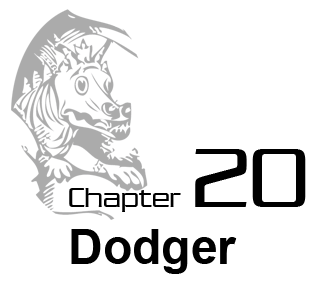
Topics Covered In This Chapter:
- The pygame.FULLSCREEN flag
- Pygame Constant Variables for Keyboard Keys
- The move_ip() Method for Rect objects
- The pygame.mouse.set_pos() Function
- Implementing Cheat Codes in Your Games
- Modifying the Dodger Game
The last three chapters have gone over the Pygame software library and demonstrated how to use its many features. (You don't need to read those chapters before reading this chapter, though it may make this chapter easier to understand.) In this chapter, we will use that knowledge to create a graphical game with sound that receives input from the keyboard and mouse.
The Dodger game has the player control a small man (which we call the player's character) who must dodge a whole bunch of baddies that fall from the top of the screen. The longer the player can keep dodging the baddies, the higher the score they will get.
Just for fun, we will also add some cheat modes to the game. If the player holds down the "x" key, every baddie's speed will be reduced to a super slow rate. If the player holds down the "z" key, the baddies will reverse their direction and travel up the screen instead of downwards.
Review of the Basic Pygame Data Types
Let's review some of the basic data types used in the Pygame library:
- pygame.Rect - Rect objects represent a rectangular space's location and size. The location can be determined by the Rect object's topleft attribute (or the topright, bottomleft, andbottomright attributes). These corner attributes are a tuple of integers for the X- and Y-coordinates. The size can be determined by the width and height attributes, which are integers of how many pixels long or high the rectangle area is. Rect objects have a colliderect() method to check if they are intersecting with another Rect object.
- pygame.Surface - Surface objects are areas of colored pixels. Surface objects represent a rectangular image, while Rect objects only represent a rectangular space and location. Surface objects have a blit() method that is used to draw the image on one Surface object onto another Surface object. The Surface object returned by the pygame.display.set_mode() function is special because anything drawn on that Surface object will be displayed on the user's screen.
- Remember that Surface have things drawn on them, but we cannot see this because it only exists in the computer's memory. We can only see a Surface object when it is "blitted" (that is, drawn) on the screen. This is just the same as it is with any other piece of data. If you think about it, you cannot see the string that is stored in a variable until the variable is printed to the screen.
- pygame.event.Event - The Event data type in the pygame.event module generates Event objects whenever the user provides keyboard, mouse, or another kind of input. Thepygame.event.get() function returns a list of Event objects. You can check what type of event the Event object is by checking its type attribute. QUIT, KEYDOWN, and MOUSEBUTTONUP are examples of some event types.
- pygame.font.Font - The pygame.font module has the Font data type which represent the typeface used for text in Pygame. You can create a Font object by calling thepygame.font.SysFont() constructor function. The arguments to pass are a string of the font name and an integer of the font size, however it is common to pass None for the font name to get the default system font. For example, the common function call to create a Font object is pygame.font.SysFont(None, 48).
- pygame.time.Clock - The Clock object in the pygame.time module are very helpful for keeping our games from running as fast as possible. (This is often too fast for the player to keep up with the computer, and makes the games not fun.) The Clock object has a tick() method, which we pass how many frames per second (fps) we want the game to run at. The higher the fps, the faster the game runs. Normally we use 40 fps. Notice that the pygame.time module is a different module than the time module which contains the sleep() function.
Type in the following code and save it to a file named dodger.py. This game also requires some other image and sound files which you can download from the URL http://inventwithpython.com/resources.
Dodger's Source Code
You can download this code from the URL http://inventwithpython.com/chapter20.
dodger.py
This code can be downloaded from http://inventwithpython.com/dodger.py
If you get errors after typing this code in, compare it to the book's code with the online diff tool at http://inventwithpython.com/diff or email the author at al@inventwithpython.com
This code can be downloaded from http://inventwithpython.com/dodger.py
If you get errors after typing this code in, compare it to the book's code with the online diff tool at http://inventwithpython.com/diff or email the author at al@inventwithpython.com
- import pygame, random, sys
- from pygame.locals import *
- WINDOWWIDTH = 600
- WINDOWHEIGHT = 600
- TEXTCOLOR = (255, 255, 255)
- BACKGROUNDCOLOR = (0, 0, 0)
- FPS = 40
- BADDIEMINSIZE = 10
- BADDIEMAXSIZE = 40
- BADDIEMINSPEED = 1
- BADDIEMAXSPEED = 8
- ADDNEWBADDIERATE = 6
- PLAYERMOVERATE = 5
- def terminate():
- pygame.quit()
- sys.exit()
- def waitForPlayerToPressKey():
- while True:
- for event in pygame.event.get():
- if event.type == QUIT:
- terminate()
- if event.type == KEYDOWN:
- if event.key == K_ESCAPE: # pressing escape quits
- terminate()
- return
- def playerHasHitBaddie(playerRect, baddies):
- for b in baddies:
- if playerRect.colliderect(b['rect']):
- return True
- return False
- def drawText(text, font, surface, x, y):
- textobj = font.render(text, 1, TEXTCOLOR)
- textrect = textobj.get_rect()
- textrect.topleft = (x, y)
- surface.blit(textobj, textrect)
- # set up pygame, the window, and the mouse cursor
- pygame.init()
- mainClock = pygame.time.Clock()
- windowSurface = pygame.display.set_mode((WINDOWWIDTH, WINDOWHEIGHT))
- pygame.display.set_caption('Dodger')
- pygame.mouse.set_visible(False)
- # set up fonts
- font = pygame.font.SysFont(None, 48)
- # set up sounds
- gameOverSound = pygame.mixer.Sound('gameover.wav')
- pygame.mixer.music.load('background.mid')
- # set up images
- playerImage = pygame.image.load('player.png')
- playerRect = playerImage.get_rect()
- baddieImage = pygame.image.load('baddie.png')
- # show the "Start" screen
- drawText('Dodger', font, windowSurface, (WINDOWWIDTH / 3), (WINDOWHEIGHT / 3))
- drawText('Press a key to start.', font, windowSurface, (WINDOWWIDTH / 3) - 30, (WINDOWHEIGHT / 3) + 50)
- pygame.display.update()
- waitForPlayerToPressKey()
- topScore = 0
- while True:
- # set up the start of the game
- baddies = []
- score = 0
- playerRect.topleft = (WINDOWWIDTH / 2, WINDOWHEIGHT - 50)
- moveLeft = moveRight = moveUp = moveDown = False
- reverseCheat = slowCheat = False
- baddieAddCounter = 0
- pygame.mixer.music.play(-1, 0.0)
- while True: # the game loop runs while the game part is playing
- score += 1 # increase score
- for event in pygame.event.get():
- if event.type == QUIT:
- terminate()
- if event.type == KEYDOWN:
- if event.key == ord('z'):
- reverseCheat = True
- if event.key == ord('x'):
- slowCheat = True
- if event.key == K_LEFT or event.key == ord('a'):
- moveRight = False
- moveLeft = True
- if event.key == K_RIGHT or event.key == ord('d'):
- moveLeft = False
- moveRight = True
- if event.key == K_UP or event.key == ord('w'):
- moveDown = False
- moveUp = True
- if event.key == K_DOWN or event.key == ord('s'):
- moveUp = False
- moveDown = True
- if event.type == KEYUP:
- if event.key == ord('z'):
- reverseCheat = False
- score = 0
- if event.key == ord('x'):
- slowCheat = False
- score = 0
- if event.key == K_ESCAPE:
- terminate()
- if event.key == K_LEFT or event.key == ord('a'):
- moveLeft = False
- if event.key == K_RIGHT or event.key == ord('d'):
- moveRight = False
- if event.key == K_UP or event.key == ord('w'):
- moveUp = False
- if event.key == K_DOWN or event.key == ord('s'):
- moveDown = False
- if event.type == MOUSEMOTION:
- # If the mouse moves, move the player where the cursor is.
- playerRect.move_ip(event.pos[0] - playerRect.centerx, event.pos[1] - playerRect.centery)
- # Add new baddies at the top of the screen, if needed.
- if not reverseCheat and not slowCheat:
- baddieAddCounter += 1
- if baddieAddCounter == ADDNEWBADDIERATE:
- baddieAddCounter = 0
- baddieSize = random.randint(BADDIEMINSIZE, BADDIEMAXSIZE)
- newBaddie = {'rect': pygame.Rect(random.randint(0, WINDOWWIDTH-baddieSize), 0 - baddieSize, baddieSize, baddieSize),
- 'speed': random.randint(BADDIEMINSPEED, BADDIEMAXSPEED),
- 'surface':pygame.transform.scale(baddieImage, (baddieSize, baddieSize)),
- }
- baddies.append(newBaddie)
- # Move the player around.
- if moveLeft and playerRect.left > 0:
- playerRect.move_ip(-1 * PLAYERMOVERATE, 0)
- if moveRight and playerRect.right < WINDOWWIDTH:
- playerRect.move_ip(PLAYERMOVERATE, 0)
- if moveUp and playerRect.top > 0:
- playerRect.move_ip(0, -1 * PLAYERMOVERATE)
- if moveDown and playerRect.bottom < WINDOWHEIGHT:
- playerRect.move_ip(0, PLAYERMOVERATE)
- # Move the mouse cursor to match the player.
- pygame.mouse.set_pos(playerRect.centerx, playerRect.centery)
- # Move the baddies down.
- for b in baddies:
- if not reverseCheat and not slowCheat:
- b['rect'].move_ip(0, b['speed'])
- elif reverseCheat:
- b['rect'].move_ip(0, -5)
- elif slowCheat:
- b['rect'].move_ip(0, 1)
- # Delete baddies that have fallen past the bottom.
- for b in baddies[:]:
- if b['rect'].top > WINDOWHEIGHT:
- baddies.remove(b)
- # Draw the game world on the window.
- windowSurface.fill(BACKGROUNDCOLOR)
- # Draw the score and top score.
- drawText('Score: %s' % (score), font, windowSurface, 10, 0)
- drawText('Top Score: %s' % (topScore), font, windowSurface, 10, 40)
- # Draw the player's rectangle
- windowSurface.blit(playerImage, playerRect)
- # Draw each baddie
- for b in baddies:
- windowSurface.blit(b['surface'], b['rect'])
- pygame.display.update()
- # Check if any of the baddies have hit the player.
- if playerHasHitBaddie(playerRect, baddies):
- if score > topScore:
- topScore = score # set new top score
- break
- mainClock.tick(FPS)
- # Stop the game and show the "Game Over" screen.
- pygame.mixer.music.stop()
- gameOverSound.play()
- drawText('GAME OVER', font, windowSurface, (WINDOWWIDTH / 3), (WINDOWHEIGHT / 3))
- drawText('Press a key to play again.', font, windowSurface, (WINDOWWIDTH / 3) - 80, (WINDOWHEIGHT / 3) + 50)
- pygame.display.update()
- waitForPlayerToPressKey()
- gameOverSound.stop()
When you run this program, the game will look like this:
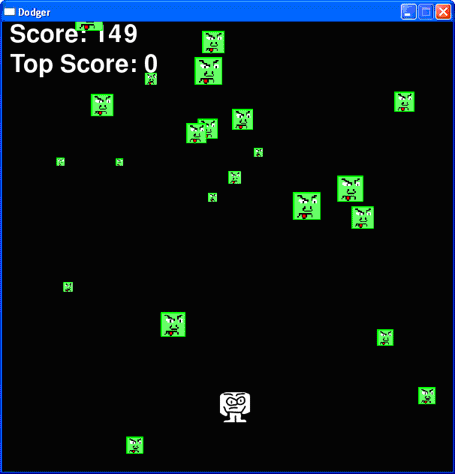
Figure 20-1: A screenshot of the Dodger game in action.
Importing the Modules
- import pygame, random, sys
- from pygame.locals import *
The Dodger game will import the same modules that our previous Pygame games have: pygame, random, sys, and pygame.locals. The pygame.locals module contains several constant variables that the Pygame library uses such as the event types (QUIT, KEYDOWN, etc.) and keyboard keys (K_ESCAPE, K_LEFT, etc.). By using the from pygame.locals import * syntax, we can just type QUITinstead of pygame.locals.QUIT.
Setting Up the Constant Variables
There are several constant variables in this game. We use constant variables because the variable name is much more descriptive than a number. For example, from the linewindowSurface.fill(BACKGROUNDCOLOR) we know that the argument being sent is a color for the background. However, the line windowSurface.fill(BACKGROUNDCOLOR) is not as clear what the argument being passed means.
We can also easily change some simple aspects about our game without having the change much of the code by changing the values stored in these constant variables. By changing WINDOWWIDTH on line 4, we automatically change the code everywhere WINDOWWIDTH is used. If we had used the value 600 instead, then we would have to change each occurrence of 600 in the code. This would be especially confusing because600 would also be used for the height of the window as well, and we would not want to change those values.
- WINDOWWIDTH = 600
- WINDOWHEIGHT = 600
- TEXTCOLOR = (255, 255, 255)
- BACKGROUNDCOLOR = (0, 0, 0)
Here we set the height and width of the main window. Since the rest of our code works off of these constant variables, changing the value here will change it everywhere in our program.
Instead of storing color tuples into a variable named WHITE or BLACK, we will use constant variables for the color of the text and background. Remember that the three integers in the color tuples range from 0 to 255and stand for red, green, and blue.
- FPS = 40
Just so the computer does not run the game too fast for the user to handle, we will call mainClock.tick() on each iteration of the game loop to slow it down. We need to pass an integer tomainClock.tick() so that the function knows how long to pause the program. This integer will be the number of frames per second we want the game to run. A "frame" is the drawing of graphics on the screen for a single iteration through the game loop. We will set up a constant variable FPS to 40, and always call mainClock.tick(FPS). You can change FPS to a higher value to have the game run faster or a lower value to slow the game down.
- BADDIEMINSIZE = 10
- BADDIEMAXSIZE = 40
- BADDIEMINSPEED = 1
- BADDIEMAXSPEED = 8
- ADDNEWBADDIERATE = 6
Here we set some more constant variables that will describe the falling baddies. The width and height of the baddies will be between BADDIEMINSIZE and BADDIEMAXSIZE. The rate at which the baddies fall down the screen will be between BADDIEMINSPEED and BADDIEMAXSPEED pixels per iteration through the game loop. And a new baddie will be added to the top of the window every ADDNEWBADDIERATEiterations through the game loop.
- PLAYERMOVERATE = 5
The PLAYERMOVERATE will store the number of pixels the player's character moves in the window on each iteration through the game loop (if the character is moving). By increasing this number, you can increase the speed the character moves. If you set PLAYERMOVERATE to 0, then the player's character won't be able to move at all (the player would move 0 pixels per iteration). This wouldn't be a very fun game.
Defining Functions
We will create several functions for our game. By putting code into functions, we can avoid having to type the same code several times in our program. And because the code is in one place, if we find a bug the code only needs to be fixed in one place.
- def terminate():
- pygame.quit()
- sys.exit()
There are several places in our game that we want to terminate the program. In our other programs, this just required a single call to sys.exit(). But since Pygame requires that we call both pygame.quit()and sys.exit(), we will put them into a function called terminate() and just call the function. This keeps us from repeating the same code over and over again. And remember, the more we type, the more likely we will make a mistake and create a bug in our program.
- def waitForPlayerToPressKey():
- while True:
- for event in pygame.event.get():
There are also a couple places where we want the game to pause and wait for the player to press a key. We will create a new function called waitForPlayerToPressKey() to do this. Inside this function, we have an infinite loop that only breaks when a KEYDOWN or QUIT event is received. At the start of the loop, we call pygame.event.get() to return a list of Event objects to check out.
- if event.type == QUIT:
- terminate()
If the player has closed the window while the program is waiting for the player to press a key, Pygame will generate a QUIT event and we should terminate the program. We will call our terminate() function here, rather than call pygame.quit() and sys.exit() themselves.
- if event.type == KEYDOWN:
- if event.key == K_ESCAPE: # pressing escape quits
- terminate()
- return
If we receive a KEYDOWN event, then we should first check if it is the Esc key that was pressed. If we are waiting for the player to press a key, and the player presses the Esc key, we want to terminate the program. If that wasn't the case, then execution will skip the if-block on line 27 and go straight to the return statement, which exits the waitForPlayerToPressKey() function.
If a QUIT or KEYDOWN event is not generated, then this loop will keep looping until it is. This will freeze the game until the player presses a key or closes the window.
- def playerHasHitBaddie(playerRect, baddies):
- for b in baddies:
- if playerRect.colliderect(b['rect']):
- return True
- return False
We will also define a function named playerHasHitBaddie() which will return True if the player's character has collided with one of the baddies. The baddies parameter is a list of baddie data structures. These data structures are just dictionaries, so it is accurate to say that baddies is a list of dictionary objects. Each of these dictionaries has a 'rect' key, and the value for that key is a Rect object that represents the baddie's size and location.
playerRect is also a Rect object. Remember that Rect objects have a method named colliderect() that returns True if the Rect object has collided with the Rect object that is passed to the method. Otherwise, colliderect() will return False.
We can use this method in our playerHasHitBaddie() function. First we iterate through each baddie data structure in the baddies list. If any of these baddies collide with the player's character, thenplayerHasHitBaddie() will return True. If the code manages to iterate through all the baddies in the baddies list without colliding with any of them, we will return False.
- def drawText(text, font, surface, x, y):
- textobj = font.render(text, 1, TEXTCOLOR)
- textrect = textobj.get_rect()
- textrect.topleft = (x, y)
- surface.blit(textobj, textrect)
Drawing text on the window involves many different steps. First, we must create a Surface object that has the string rendered in a specific font on it. The render() method does this. Next, we need to know the size and location of the Surface object we just made. We can get a Rect object with this information with the get_rect() method for Surface objects.
This Rect object has no special connection to the Surface object with the text drawn on it, other than the fact that it has a copy of the width and height information from the Surface object. We can change the location of the Rect object by setting a new tuple value for its topleft attribute.
Finally, we blit the Surface object of the rendered text onto the Surface object that was passed to our drawText() function. Displaying text in Pygame take a few more steps than simply calling the print()function, but if we put this code into a single function (drawText()), then we only need to call the function instead of typing out all the code every time we want to display text on the screen.
Initializing Pygame and Setting Up the Window
Now that the constant variables and functions are finished, we can start calling the Pygame functions that will set up Pygame for use in our code. Many of these function calls are to set up the GUI window and create objects that we will use in the game.
- # set up pygame, the window, and the mouse cursor
- pygame.init()
- mainClock = pygame.time.Clock()
- windowSurface = pygame.display.set_mode((WINDOWWIDTH, WINDOWHEIGHT))
- pygame.display.set_caption('Dodger')
- pygame.mouse.set_visible(False)
Line 43 sets up the Pygame library. Remember, the pygame.init() function must be called before we can use any of Pygame's functions or data types. Line 44 creates a pygame.time.Clock() object and stores it in the mainClock variable. This object will help us keep the program from running too fast.
Line 45 creates a new Surface object which will be used for the window displayed on the screen. We will specify the width and height of this Surface object (and the window) by passing a tuple with theWINDOWWIDTH and WINDOWHEIGHT constant variables. Notice that there is only one argument passed to pygame.display.set_mode(): a tuple. The arguments forpygame.display.set_mode() are not two integers but a tuple of two integers.
On line 46, the caption of the window is set to the string 'Dodger'. This caption will appear in the title bar at the top of the window.
In our game, we do not want the mouse cursor (the mouse cursor is the arrow that moves around the screen when we move the mouse) to be visible. This is because we want the mouse to be able to move the player's character around the screen, and the arrow cursor would get in the way of the character's image on the screen. We pass False to tell Pygame to make the cursor invisible. If we wanted to make the cursor visible again at some point in the program, we could call pygame.mouse.set_visible(True).
Fullscreen Mode
The pygame.display.set_mode() function has a second, optional parameter that you can pass to it. The value you can pass for this parameter is pygame.FULLSCREEN, like this modification to line 45 in our Dodger program:
- windowSurface = pygame.display.set_mode((WINDOWWIDTH, WINDOWHEIGHT), pygame.FULLSCREEN)
Passing pygame.FULLSCREEN will make the program take up the entire space of the screen. It will still be WINDOWWIDTH and WINDOWHEIGHT in size for the windows width and height, but the image will be stretched larger to fit the screen. There may be wasted space along the top and bottom (or the left and right) sides of the screen if you did not set the window size in proportion with the screen's resolution.) To avoid the wasted space, you should set the size of the window to a 4:3 ratio (for every 4 pixels of width, have 3 pixels for height).
If you do not use the fullscreen mode, then you do not need to worry about using a 4:3 ratio for the width and height. Just use whatever width and height works best for your game.
- # set up fonts
- font = pygame.font.SysFont(None, 48)
We need to create a Font object to use when we create a Surface object with the image of text drawn on it. (This process is called "rendering".) We want to create a generic font, so we will use the default Fontobject that the pygame.font.SysFont() constructor function returns. We pass None so that the default font is used, and we pass 48 so that the font has a size of 48 points.
- # set up sounds
- gameOverSound = pygame.mixer.Sound('gameover.wav')
- pygame.mixer.music.load('background.mid')
Next we want to create the Sound objects and also set up the background music. The background music will constantly be playing during the game, but Sound objects will only be played when we specifically want them to. In this case, the Sound object will be played when the player loses the game.
You can use any .wav or .mid file for this game. You can download these sound files from this book's website at the URL http://inventwithpython.com/resources. Or you can use your own sound files for this game, as long as they have the filenames of gameover.wav and background.mid. (Or you can change the strings used on lines 53 and 54 to match the filenames.)
The pygame.mixer.Sound() constructor function creates a new Sound object and stores a reference to this object in the gameOverSound variable. In your own games, you can create as many Soundobjects as you like, each with a different sound file that it will play.
The pygame.mixer.music.load() function loads a sound file to play for the background music. This function does not create any objects, and only one sound file can be loaded at a time.
- # set up images
- playerImage = pygame.image.load('player.png')
- playerRect = playerImage.get_rect()
- baddieImage = pygame.image.load('baddie.png')
Next we will load the image files that used for the player's character and the baddies on the screen. The image for the character is stored in player.png and the image for the baddies is stored in baddie.png. All the baddies look the same, so we only need one image file for them. You can download these images from the book's website at the URL http://inventwithpython.com/resources.
Display the Start Screen
When the game first starts, we want to display the name of the game on the screen. We also want to instruct the player that they can start the game by pushing any key. This screen appears so that the player has time to get ready to start playing after running the program. Also, before each game starts, we want to reset the value of the top score back to 0.
- # show the "Start" screen
- drawText('Dodger', font, windowSurface, (WINDOWWIDTH / 3), (WINDOWHEIGHT / 3))
- drawText('Press a key to start.', font, windowSurface, (WINDOWWIDTH / 3) - 30, (WINDOWHEIGHT / 3) + 50)
- pygame.display.update()
- waitForPlayerToPressKey()
On lines 62 and 63, we call our drawText() function and pass it five arguments: 1) the string of the text we want to appear, 2) the font that we want the string to appear in, 3) the Surface object onto which to render the text, and 4) and 5) the X and Y coordinate on the Surface object to draw the text at.
This may seem like many arguments to pass for a function call, but keep in mind that this function call replaces five lines of code each time we call it. This shortens our program and makes it easier to find bugs since there is less code to check.
The waitForPlayerToPressKey() function will pause the game by entering into a loop that checks for any KEYDOWN events. Once a KEYDOWN event is generated, the execution breaks out of the loop and the program continues to run.
Start of the Main Game Code
- topScore = 0
- while True:
We have finished defining the helper functions and variables that we need for this game. Line 68 is the start of the main game code. The value in the topScore variable starts at 0 only when the program first runs. Whenever the player loses and has a score larger than the current top score, the top score is replaced with the player's score.
The infinite loop started on line 69 is technically not the "game loop". (The main game loop handles events and drawing the window while the game is running.) Instead, this while loop will iterate each time the player starts a new game. We will set up the code so that when the player loses and we need to reset the game, the program's execution will go back to the start of this loop.
- # set up the start of the game
- baddies = []
- score = 0
At the very beginning, we want to set the baddies list to an empty list. The baddies list is a list of dictionary objects with the following keys:
- 'rect' - The Rect object that describes where and what size the baddie is.
- 'speed' - How fast the baddie falls down the screen. This integer represents pixels per iteration through the game loop.
- 'surface' - The Surface object that has the scaled image of the baddie image drawn on it. This is the Surface object that will be blitted to the Surface object returned bypygame.display.set_mode() and drawn on the screen.
Next, we want to reset the player's score to 0.
- playerRect.topleft = (WINDOWWIDTH / 2, WINDOWHEIGHT - 50)
The starting location of the player will be in the center of the screen and 50 pixels up from the bottom. The tuple that we set the topleft attribute to will change the location of the playerRect object. The first item in the tuple is the X-coordinate of the left edge. The second item in the tuple is the Y-coordinate of the top edge.
- moveLeft = moveRight = moveUp = moveDown = False
- reverseCheat = slowCheat = False
- baddieAddCounter = 0
Also at the start of the game, we want to have the movement variables moveLeft, moveRight, moveUp, and moveDown set to False. The reverseCheat and slowCheat variables will be set toTrue only when the player enables these cheats by holding down the "z" and "x" keys, respectively.
The baddieAddCounter variable is used for a counter to tell the program when to add a new baddie at the top of the screen. The value in baddieAddCounter will be incremented by one each time the game loop iterates. When the baddieAddCounter counter is equal to the value in ADDNEWBADDIERATE, then the baddieAddCounter counter is reset back to 0 and a new baddie is added to the top of the screen.
- pygame.mixer.music.play(-1, 0.0)
At the start of the game, we want the background music to begin playing. We can do this with a call to pygame.mixer.music.play(). The first argument is the number of times the music should repeat itself. -1 is a special value that tells Pygame we want the music to repeat endlessly. The second argument is a float that says how many seconds into the music we want it to start playing. Passing 0.0 means we want to play the music starting from the beginning of the music file. (Passing 2.0, for example, would have started the music two seconds into the music file.)
The Game Loop
The game loop contains the code that is executed while the game is being played. The game loop constantly updates the state of the game world by changing the position of the player and baddies, handling events generated by Pygame, and drawing the state of the game world on the screen. All of this happens several dozen times a second, which makes it seem that the game is happening in real time to the player.
- while True: # the game loop runs while the game part is playing
- score += 1 # increase score
Line 79 is the start of the main game loop. In the main game loop, we will increase the player's score, handle any events that were generated, add any baddies to the top of the screen if needed, move the baddies down a little, and then draw everything on the screen. This code will be executed over and over again as the program execution iterates through the game loop. The loop will only exit when the player either loses the game or quits the program.
First, we will increment the player's score. The longer the player can go without losing, the higher their score will be.
Event Handling
There are four different types of events we will handle in our game: QUIT, KEYDOWN, KEYUP, and MOUSEMOTION. The QUIT event is generated by Pygame if the player closes the program's window or shuts down the computer. In that case, we want the program to close itself. The KEYDOWN and KEYUP events are generated when the player pushes down and releases the keyboard keys, respectively. These events will be how we can tell which direction the player wants to move the character. The player could also have pressed the Esc key to signal that they want to shut down the program. Each time the player moves the mouse, Pygame will generate a MOUSEMOTION event which will tell us the X- and Y-coordinates of the mouse cursor over the window.
- for event in pygame.event.get():
- if event.type == QUIT:
- terminate()
Line 82 is the start of the event-handling code. First we call pygame.event.get(), which returns a list of Event objects. Each Event object represents an event that has been created since the last call topygame.event.get(). We will check the type attribute of the event object to see what type of event it is, and handle the event accordingly.
If the type attribute of the Event object is equal to QUIT, then this tells us that the user has closed the program somehow. The QUIT constant variable was imported from the pygame.locals module, but since we imported that module with the line from pygame.locals import * instead of simply import pygame.locals, we only need to type QUIT and not pygame.locals.QUIT.
- if event.type == KEYDOWN:
- if event.key == ord('z'):
- reverseCheat = True
- if event.key == ord('x'):
- slowCheat = True
If the event's type is KEYDOWN, then we know that the player has pressed down a key. The Event object for keyboard events will also have a key attribute that is set to the numeric ASCII value of the key pressed. The ord() function will return the ASCII value of the letter passed to it.
For example, on line 87, we can check if the event describes the "z" key being pressed down by checking if event.key == ord('z'). If this condition is True, then we want to set the reverseCheatvariable to True to indicate that the reverse cheat has been activated. We will also check if the "x" key has been pressed to activate the slow cheat in a similar way.
Pygame's keyboard events always use the ASCII values of lowercase letters, not uppercase. What this means for your code is that you should always use event.key == ord('z') instead of event.key == ord('Z'). Otherwise, your program may act as though the key hasn't been pressed at all.
- if event.key == K_LEFT or event.key == ord('a'):
- moveRight = False
- moveLeft = True
- if event.key == K_RIGHT or event.key == ord('d'):
- moveLeft = False
- moveRight = True
- if event.key == K_UP or event.key == ord('w'):
- moveDown = False
- moveUp = True
- if event.key == K_DOWN or event.key == ord('s'):
- moveUp = False
- moveDown = True
We also want to check if the event was generated by the player pressing one of the arrow keys. There is not an ASCII value for every key on the keyboard, such as the arrow keys or the Esc key. Instead, Pygame provides some constant variables to use instead.
We can check if the player has pressed the left arrow key with the condition: event.key == K_LEFT. Again, the reason we can use K_LEFT instead of pygame.locals.K_LEFT is because we importedpygame.locals with the line from pygame.locals import * instead of import pygame.locals.
Noticed that pressing down on one of the arrow keys not only sets one of the movement variables to True, but it also sets the movement variable in the opposite direction to False. For example, if the left arrow key is pushed down, then the code on line 93 sets moveLeft to True, but it also sets moveRight to False. This prevents the player from confusing the program into thinking that the player's character should move in two opposite directions at the same time.
Here is a list of commonly-used constant variables for the key attribute of keyboard-related Event objects:
Pygame Constant Variable | Keyboard Key | Pygame Constant Variable | Keyboard Key |
---|---|---|---|
K_LEFT | Left arrow | K_HOME | Home |
K_RIGHT | Right arrow | K_END | End |
K_UP | Up arrow | K_PAGEUP | PgUp |
K_DOWN | Down arrow | K_PAGEDOWN | PgDn |
K_ESCAPE | Esc | K_F1 | F1 |
K_BACKSPACE | Backspace | K_F2 | F2 |
K_TAB | Tab | K_F3 | F3 |
K_RETURN | Return or Enter | K_F4 | F4 |
K_SPACE | Space bar | K_F5 | F5 |
K_DELETE | Del | K_F6 | F6 |
K_LSHIFT | Left Shift | K_F7 | F7 |
K_RSHIFT | Right Shift | K_F8 | F8 |
K_LCTRL | Left Ctrl | K_F9 | F9 |
K_RCTRL | Right Ctrl | K_F10 | F10 |
K_LALT | Left Alt | K_F11 | F11 |
K_RALT | Right Alt | K_F12 | F12 |
- if event.type == KEYUP:
- if event.key == ord('z'):
- reverseCheat = False
- score = 0
- if event.key == ord('x'):
- slowCheat = False
- score = 0
The KEYUP event is created whenever the player stops pressing down on a keyboard key and it returns to its normal, up position. KEYUP objects with a type of KEYUP also have a key attribute just like KEYDOWNevents.
On line 105, we check if the player has released the "z" key, which will deactivate the reverse cheat. In that case, we set reverseCheat to False and reset the score to 0. The score reset is to discourage the player for using the cheats.
Lines 108 to 110 do the same thing for the "x" key and the slow cheat. When the "x" key is released, slowCheat is set to False and the player's score is reset to 0.
- if event.key == K_ESCAPE:
- terminate()
At any time during the game, the player can press the Esc key on the keyboard to quit the game. Here we check if the key that was released was the Esc key by checking event.key == K_ESCAPE. If so, we call our terminate() function which will exit the program.
- if event.key == K_LEFT or event.key == ord('a'):
- moveLeft = False
- if event.key == K_RIGHT or event.key == ord('d'):
- moveRight = False
- if event.key == K_UP or event.key == ord('w'):
- moveUp = False
- if event.key == K_DOWN or event.key == ord('s'):
- moveDown = False
Lines 114 to 121 check if the player has stopped holding down one of the arrow keys (or the corresponding WASD key). In that event, we will set the corresponding movement variable to False. For example, if the player was holding down the left arrow key, then the moveLeft would have been set to True on line 93. When they release it, the condition on line 114 will evaluate to True, and the moveLeft variable will be set to False.
The move_ip() Method for Rect objects
- if event.type == MOUSEMOTION:
- # If the mouse moves, move the player where the cursor is.
- playerRect.move_ip(event.pos[0] - playerRect.centerx, event.pos[1] - playerRect.centery)
Now that we have handled the keyboard events, let's handle any mouse events that may have been generated. In the Dodger game we don't do anything if the player has clicked a mouse button, but the game does respond when the player moves the mouse. This gives the player two ways of controlling the player character in the game: the keyboard and the mouse.
If the event's type is MOUSEMOTION, then we want to move the player's character to the location of the mouse cursor. The MOUSEMOTION event is generated whenever the mouse is moved. Event objects with atype of MOUSEMOTION also have an attribute named pos. The pos attribute stores a tuple of the X- and Y-coordinates of where the mouse cursor moved in the window.
The move_ip() method for Rect objects will move the location of the Rect object horizontally or vertically by a number of pixels. For example, playerRect.move_ip(10, 20) would move the Rectobject 10 pixels to the right and 20 pixels down. To move the Rect object left or up, pass negative values. For example, playerRect.move_ip(-5, -15) will move the Rect object left by 5 pixels and up 15 pixels.
The "ip" at the end of move_ip() stands for "in place". This is because the method changes the Rect object itself, in its own place. There is also a move() method which does not change the Rect object, but instead creates a new Rect object that has the new location. This is useful if you want to keep the original Rect object's location the same but also have a Rect object with the new location.
Adding New Baddies
- # Add new baddies at the top of the screen, if needed.
- if not reverseCheat and not slowCheat:
- baddieAddCounter += 1
On each iteration of the game loop, we want to increment the baddieAddCounter variable by one. However, we only want to do this if the cheats are not enabled. Remember that reverseCheat andslowCheat: are only set to True as long as the "z" and "x" keys are being held down, respectively. And while those keys are being held down, baddieAddCounter is not incremented. This means that no new baddies will appear at the top of the screen.
- if baddieAddCounter == ADDNEWBADDIERATE:
- baddieAddCounter = 0
- baddieSize = random.randint(BADDIEMINSIZE, BADDIEMAXSIZE)
- newBaddie = {'rect': pygame.Rect(random.randint(0, WINDOWWIDTH-baddieSize), 0 - baddieSize, baddieSize, baddieSize),
- 'speed': random.randint(BADDIEMINSPEED, BADDIEMAXSPEED),
- 'surface':pygame.transform.scale(baddieImage, (baddieSize, baddieSize)),
- }
When the baddieAddCounter reaches the value in ADDNEWBADDIERATE, then the condition on line 130 is True and it is time to add a new baddie to the top of the screen. First, thebaddieAddCounter counter is reset back to 0 (otherwise, when it keeps incrementing it will always be greater than ADDNEWBADDIERATE and never equal to it. This will cause baddies to stop appearing at the top of the screen.)
Line 132 generates a size for the baddie in pixels. The size will be between BADDIEMINSIZE and BADDIEMAXSIZE, which we have set to 10 and 40 in this program.
Line 133 is where a new baddie data structure is created. Remember, the data structure for baddies is simply a dictionary with keys 'rect', 'speed', and 'surface'. The 'rect' key holds a reference to aRect object which stores the location and size of the baddie. The call to the pygame.Rect() constructor function has four parameters: the X-coordinate of the top edge of the area, the Y-coordinate of the left edge of the area, the width in pixels, and the height in pixels.
We want the baddie to appear randomly across the top of the window, so we pass random.randint(0, WINDOWWIDTH-baddieSize) for the X-coordinate of the left edge. This will evaluate to a random place across the top of the window. The reason we pass WINDOWWIDTH-baddieSize instead of WINDOWWIDTH is because this value is for the left edge of the baddie. If the left edge of the baddie is too far on the right side of the screen, then part of the baddie will be off the edge of the window and not visible.
We want the bottom edge of the baddie to be just above the top edge of the window. The Y-coordinate of the top edge of the window is 0, so to put the baddie's bottom edge there, we want to set the top edge to 0 - baddieSize.
The baddie's width and height should be the same (the image is a square), so we will pass baddieSize for the third and fourth argument.
The rate of speed that the baddie moves down the screen will be set in the 'speed' key, and is set to a random integer between BADDIEMINSPEED and BADDIEMAXSPEED.
- baddies.append(newBaddie)
Line 138 will add the newly created baddie data structure to the list of baddie data structures. Our program will use this list to check if the player has collided with any of the baddies and to know where to draw baddies on the window.
Moving the Player's Character
- # Move the player around.
- if moveLeft and playerRect.left > 0:
- playerRect.move_ip(-1 * PLAYERMOVERATE, 0)
The four movement variables moveLeft, moveRight, moveUp and moveDown are set to True and False when Pygame generates the KEYDOWN and KEYUP events, respectively. (This code is from line 86 to line 121.)
If the player's character is moving left and the left edge of the player's character is greater than 0 (which is the left edge of the window), then we want to move the character's Rect object (stored in playerRect).
We will always move the playerRect object by the number of pixels in PLAYERMOVERATE. To get the negative form of an integer, you can simply multiple it by -1. So on line 142, since 5 is stored inPLAYERMOVERATE, the expression -1 * PLAYERMOVERATE evaluates to -5.
This means that calling playerRect.move_ip(-1 * PLAYERMOVERATE, 0) will change the location of playerRect by 5 pixels to the left of its current location.
- if moveRight and playerRect.right < WINDOWWIDTH:
- playerRect.move_ip(PLAYERMOVERATE, 0)
- if moveUp and playerRect.top > 0:
- playerRect.move_ip(0, -1 * PLAYERMOVERATE)
- if moveDown and playerRect.bottom < WINDOWHEIGHT:
- playerRect.move_ip(0, PLAYERMOVERATE)
We want to do the same thing for the other three directions: right, up, and down. Each of the three if statements in lines 143 to 148 checks that their movement variable is set to True and that the edge of the Rectobject of the player is inside the window before calling the move_ip() method to move the Rect object.
The pygame.mouse.set_pos() Function
- # Move the mouse cursor to match the player.
- pygame.mouse.set_pos(playerRect.centerx, playerRect.centery)
Line 151 moves the mouse cursor to the same position as the player's character. The pygame.mouse.set_pos() function moves the mouse cursor to the X- and Y-coordinates that you pass it. Specifically, the cursor will be right in the middle of the character's Rect object because we pass the centerx and centery attributes of playerRect for the coordinates. The mouse cursor still exists and can be moved, even though it is invisible because we called pygame.mouse.set_visible(False) on line 47.
The reason we want the mouse cursor to match the location of the player's character is to avoid sudden jumps. Imagine that the mouse cursor and the player's character are at the same location on the left side of the window. When the player holds down the right arrow key, the character moves to the right edge of the window but the mouse cursor would stay at the left edge of the screen. If the player then moves the mouse just a little bit, the player's character would immediately jump to the location of the mouse cursor on the left edge of the screen. By moving the mouse cursor along with the player's character, any mouse movements would not result in a sudden jump across the window.
- # Move the baddies down.
- for b in baddies:
Now we want to loop through each baddie data structure in the baddies list to move them down a little.
- if not reverseCheat and not slowCheat:
- b['rect'].move_ip(0, b['speed'])
If neither of the cheats have been activated (by the player pushing the "z" or "x" keys which sets reverseCheat or slowCheat to True, respectively), then move the baddie's location down a number of pixels equal to its speed, which is stored in the 'speed' key.
Implementing the Cheat Codes
- elif reverseCheat:
- b['rect'].move_ip(0, -5)
If the reverse cheat has been activated, then the baddie should actually be moved up by five pixels. Passing -5 for the second argument to move_ip() will move the Rect object upwards by five pixels.
- elif slowCheat:
- b['rect'].move_ip(0, 1)
If the slow cheat has been activated, then the baddie should move downwards, but only by the slow speed of one pixel per iteration through the game loop. The baddie's normal speed (which is stored in the 'speed'key of the baddie's data structure) will be ignored while the slow cheat is activated.
Removing the Baddies
- # Delete baddies that have fallen past the bottom.
- for b in baddies[:]:
After moving the baddies down the window, we want to remove any baddies that fell below the bottom edge of the window from the baddies list. Remember that we while we are iterating through a list, we should not modify the contents of the list by adding or removing items. So instead of iterating through the baddies list with our baddies loop, we will iterate through a copy of the baddies list.
Remember that a list slice will evaluate a copy of a list's items. For example, spam[2:4] will return a new list with the items from index 2 up to (but not including) index 4. Leaving the first index blank will indicate that index 0 should be used. For example, spam[:4] will return a list with items from the start of the list up to (but not including) the item at index 4. Leaving the second index blank will indicate that up to (and including) the last index should be used. For example, spam[2:] will return a list with items from index 2 all the way to (and including) the last item in the list.
But leaving both indexes in the slice blank is a way to represent the entire list. The baddies[:] expression is a list slice of the whole list, so it evaluates to a copy of the entire list. This is useful because while we are iterating on the copy of the list, we can modify the original list and remove any baddie data structures that have fallen past the bottom edge of the window.
Our for loop on line 163 uses a variable b for the current item in the iteration through baddies[:].
- if b['rect'].top > WINDOWHEIGHT:
- baddies.remove(b)
Let's evaluate the expression b['rect'].top. b is the current baddie data structure from the baddies[:] list. Each baddie data structure in the list is a dictionary with a 'rect' key, which stores a Rectobject. So b['rect'] is the Rect object for the baddie. Finally, the top is the Y-coordinate of the top edge of the rectangular area. Remember that in the coordinate system, the Y-coordinates increase going down. Sob['rect'].top > WINDOWHEIGHT will check if the top edge of the baddie is below the bottom of the window.
If this condition is True, then the we will remove the baddie data structure from the baddies list.
Drawing the Window
It isn't enough that our game updates the state of the game world in its memory. Our program will also have to display the game world to the player. We can do this by drawing the graphics of the baddies and player's character on the screen. Because the game loop is executed several times a second, drawing the baddies and player in new positions makes their movement look smooth and natural. But every element on the screen must be drawn one at a time by calling the appropriate Pygame function.
- # Draw the game world on the window.
- windowSurface.fill(BACKGROUNDCOLOR)
Now that we have updated all the data structures for the baddies and the player's character, let's draw everything on the screen. First, before we draw anything else on the Surface object referred to bywindowSurface, we want to black out the entire screen to erase anything drawn on it in a previous iteration through the game loop.
Remember that the Surface object in windowSurface is the special Surface object because it was the one returned by pygame.display.set_mode(). This means that anything drawn on thatSurface object will appear on the screen, but only after the pygame.display.update() function is called.
Drawing the Player's Score
- # Draw the score and top score.
- drawText('Score: %s' % (score), font, windowSurface, 10, 0)
- drawText('Top Score: %s' % (topScore), font, windowSurface, 10, 40)
Next we will render the text for score and top score to the top left corner of the window. The 'Score: %s' % (score) uses string interpolation to insert the value in the score variable into the string. This is the same thing as 'Score: ' + str(score). We pass this string, the Font object stored in the font variable, the Surface object on which to draw the text on, and the X- and Y-coordinates of where the text should be placed. Remember that our drawText() will handle the call to the render() and blit() methods.
For the top score, we do the exact same thing. We pass 40 for the Y-coordinate instead of 0 (like we do for the score) so that the top score text appears beneath the score text.
Drawing the Player's Character
- # Draw the player's rectangle
- windowSurface.blit(playerImage, playerRect)
Remember that the information about the player is kept in two different variables. playerImage is a Surface object that contains all the colored pixels that make up the player's character's image. playerRectis a Rect object that stores the information about the size and location of the player's character.
We call the blit() method on windowSurface and pass playerImage and playerRect. This draws the player character's image on windowSurface at the appropriate location.
- # Draw each baddie
- for b in baddies:
- windowSurface.blit(b['surface'], b['rect'])
We use a for loop here to draw every baddie on the windowSurface object. Remember that each item in the baddies list is a dictionary with 'surface' and 'rect' keys containing the Surfaceobject with the baddie image and the Rect object with the position and size information, respectively.
- pygame.display.update()
Now that we have finished drawing everything to the windowSurface object, we should draw this surface to the screen with a call to pygame.display.update().
Collision Detection
- # Check if any of the baddies have hit the player.
- if playerHasHitBaddie(playerRect, baddies):
- if score > topScore:
- topScore = score # set new top score
- break
Now let's check if the player has collided with any of the baddies. We already wrote a function to check for this: playerHasHitBaddie(). This function will return True if the player's character has collided with any of the baddies in the baddies list. Otherwise, the function will return False.
If the player's character has hit a baddie, then we check if the player's current score is greater than the top score. If it is, we set the new top score to be the player's current score. Either way, we break out of the game loop. The program's execution will jump down to line 191.
- mainClock.tick(FPS)
To keep the computer from running through the game loop as fast as possible (which would be much too fast for the player to keep up with), we call mainClock.tick() to pause for a brief amount of time. The pause will be long enough to ensure that about 40 (the value we stored inside the FPS variable) iterations through the game loop occur each second.
The Game Over Screen
- # Stop the game and show the "Game Over" screen.
- pygame.mixer.music.stop()
- gameOverSound.play()
When the player loses, we want to stop playing the background music and play the "game over" sound effect. We call the stop() function in the pygame.mixer.music module to stop the background music. Then we call the play() method on the Sound object stored in gameOverSound.
- drawText('GAME OVER', font, windowSurface, (WINDOWWIDTH / 3), (WINDOWHEIGHT / 3))
- drawText('Press a key to play again.', font, windowSurface, (WINDOWWIDTH / 3) - 80, (WINDOWHEIGHT / 3) + 50)
- pygame.display.update()
- waitForPlayerToPressKey()
Now we want to display text on the window to tell the player that the game is over, and they should press a key to start playing a new game. The two calls to our drawText() function will draw this text to thewindowSurface object, and the call to pygame.display.update() will draw this Surface object to the screen.
After displaying this text, we want the game to stop until the player presses a key, so we call our waitForPlayerToPressKey() function.
- gameOverSound.stop()
After the player presses a key, the program execution will return from the waitForPlayerToPressKey() call on line 198. Depending on how long the player takes to press a key, the "game over" sound effect may or may not still be playing. We want to stop this sound effect before this loop ends and we start a new game, so we have a call to gameOverSound.stop() here.
Modifying the Dodger Game
That's it for our graphical game. You may find that the game is too easy or too hard. But the game is very easy to modify because we took the time to use constant variables instead of typing in the values directly. Now all we need to do to change the game is modify the value set in the constant variables.
For example, if you want the game to run slower in general, change the FPS variable on line 8 to a smaller value such as 20. This will make both the baddies and the player's character move slower since the game loop will only be executed 20 times a second instead of 40.
If you just want to slow down the baddies and not the player, then change BADDIEMAXSPEED to a smaller value such as 4. This will make all the baddies move between 1 (the value in BADDIEMINSPEED) and 4 pixels per iteration through the game loop instead of 1 and 8.
If you want the game to have fewer but larger baddies instead of many fast baddies, then increase ADDNEWBADDIERATE to 12, BADDIEMINSIZE to 40, and BADDIEMAXSIZE to 80. Now that baddies are being added every 12 iterations through the game loop instead of every 6 iterations, there will be half as many baddies as before. But to keep the game interesting, the baddies are now much larger than before.
While the basic game remains the same, you can modify any of the constant variables to drastically affect the behavior of the game. Keep trying out new values for the constant variables until you find a set of values you like the best.
Summary: Creating Your Own Games
Unlike our previous text-based games, Dodger really looks like the kind of modern computer game we usually play. It has graphics and music and uses the mouse. While Pygame provides functions and data types as building blocks, it is you the programmer who puts them together to create fun, interactive games.
And it is all because you know exactly how to instruct the computer to do it, step by step, line by line. You can speak the computer's language, and get it to do large amounts of number crunching and drawing for you. This is a very useful skill, and I hope you will continue to learn more about Python programming. (And there is still more to learn!)
Here are several websites that can teach you more about programming Python:
- http://www.python.org/doc/ - More Python tutorials and the documentation of all the Python modules and functions.
- http://www.pygame.org/docs/ - Complete documentation on the modules and functions for Pygame.
- http://inventwithpython.com - This book's website, which includes all the source code for these programs and additional information. This site also has the image and sound files used in the Pygame programs.
- http://inventwithpython.com/traces - A web application that helps you trace through the execution of the programs in this book, step by step.
- http://inventwithpython.com/videos - Videos that accompany the programs in this book.
- http://gamedevlessons.com - A helpful website about how to design and program video games.
- al@inventwithpython.com - The author's email address. Feel free to email Al your questions about this book or about Python programming.
Or you can find out more about Python by searching the World Wide Web. Go to the search engine website http://google.com and search for "Python programming" or "Python tutorials" to find web sites that can teach you more about Python programming.
Now get going and invent your own games. And good luck!
0 comments:
Post a Comment